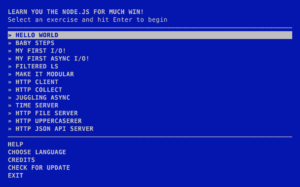
I spent the better part of a day recently working through the NodeSchool tutorial learnyounode. It’s a great introduction to Node.js, but some of the hints (and documentation) leave gaps. Answers are easier to find than additional hints, so I thought I’d type up my sticking points in the form of hints.
General Notes:
- All command-line arguments are provided by learnyounode, for both run and verify.
- Whenever you do something asynchronous, anything callback-y must be within the asynchronous function or controlled by something inside. Otherwise it will fire immediately (i.e. way too early).
Challenge 5: Filtered ls
Note that the extension input has no period but path.extname’s output includes the period.
Challenge 6: Make it Modular
If your module is module6, your main file function call should be module6(directory, extension, function(error, data){…});
module6.js will be module.exports = function(directory, extension, callback) {…}
The module function will include the fs.readdir function, which is asynchronous (see general note 2).
Challenge 7: HTTP Client
I had a reading comprehension failure on this one: although fs.readFile can take ‘utf8’ as an intermediate parameter, response.on cannot. It is a separate line.
Challenge 8: HTTP Collect
(for approach 1): ‘end’ and ‘data’ are both response events – just as you can have response(‘data’, callback) you can use response(‘end’, callback).
Challenge 9: Juggling Async
I found this one quite difficult and was saved by a blog post from The Polyglot Developer. It took a bit of translation, though, so a second hint appears after the first bank of hints.
Unrelated caution: don’t put your http.get directly inside a for loop with the loop index determining the destination of the response text – asynchronicity will make everyone write to the destination corresponding to the loop index’s final value (which is generally not somewhere you were expecting anyone to write to). You’ll need a function in between so a local (read: persistent) copy of the index is made.
Challenge 10: Time Server
Note that if a Date method is giving you a numerical value, it will be a Number, not a String.
Challenge 11: HTTP File Server
The two arguments of http.createServer’s callback function are both streams. Read the last paragraph of the included hints closely knowing that. Another hint below.
Challenge 12: HTTP Uppercaserer
The given hints for this are very thorough; I have only the hints for 11 above, plus remember to install through2-map. Also note the verifier doesn’t actually check for rejection of non-POST requests.
Challenge 13: HTTP JSON API Server
That url is a Node core module means you won’t have to install it, not that you won’t have to require it. Running the “for example, on the command prompt” line is key to solving this challenge.
Unlike in Challenge 10 you don’t need leading zeroes on single-digit time values. In fact you can’t have them because the verification will fail if your time values were Strings instead of Numbers pre-JSON-stringification.
Challenge 9, hint 2:
While your completion counter variable needs to be declared outside the get request, so all of the requests are accessing the same one, you’ll need to increment and test its value within the request.on(‘end’) event. Keep the responses in input order via a data structure for correct outputting to the console.
Challenge 11, hint 2:
You will ignore the request stream because your response pertains to any request at all. fs.createReadStream() gives you a stream, so you can call pipe() right on that.